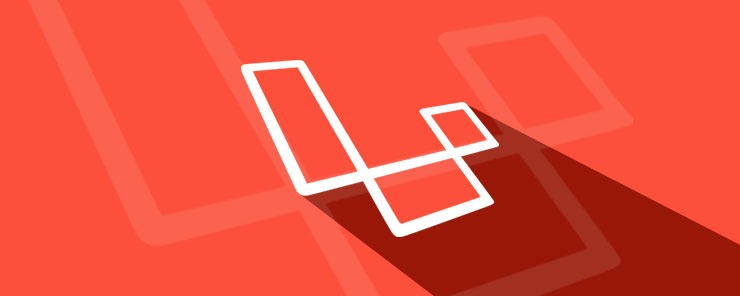
Property Class
class Property extends Model
{
protected $table = 'properties';
use HasFactory;
/**
* Get the Street that owns the Property
*
* @return \Illuminate\Database\Eloquent\Relations\BelongsTo
*/
public function street(): BelongsTo
{
return $this->belongsTo(Street::class);
}
/**
* Get the Neighbourhood that owns the Property
*
* @return \Illuminate\Database\Eloquent\Relations\BelongsTo
*/
public function neighbourhood(): BelongsTo
{
return $this->belongsTo(Neighbourhood::class);
}
}
Resident Class
class Resident extends Model
{
use HasFactory;
protected $guarded = [];
/**
* The Property that belong to the Resident
*
* @return \Illuminate\Database\Eloquent\Relations\BelongsToMany
*/
public function properties(): BelongsToMany
{
return $this->belongsToMany(Property::class);
}
}
Pivot class, PropertyResident class.
class PropertyResident extends Model
{
use HasFactory;
protected $fillable = ['property_id', 'resident_id'];
}
Use tinker to test
php artisan tinker
//To find resident id
$r=App\Models\Resident::find(1)
//To get all resident properties
$r->properties
//To count all resident properties
$r->properties->count()
//To find resident id(1) neighbourhood trough properties (many to many relationship)
$r->properties->find(5)->neighbourhood->name
//To get all resident id(1) property "property_id"
$r->properties()->pluck('property_id')
//To get distinct(*) neighbourhood
$r->properties()->distinct()->pluck('neighbourhood_id')
//To get all neighbourhood id group by neighbourhood id
$r->properties()->groupBy('neighbourhood_id')->pluck('neighbourhood_id')
Leave a Reply