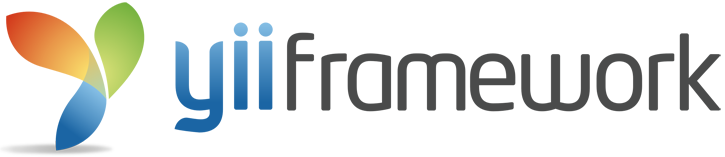
- Download this mail extension here http://www.yiiframework.com/extension/mail.
- Extract the files.
- Move the vendors folder to protected/components/vendors/
- Move YiiMail.php and YiiMailMessage.php to protected/components/
- Add on protected/config/main.php
[php]
‘components’=>array(
…
‘mail’ => array(
‘class’ => ‘YiiMail’,
‘transportType’ => ‘php’,
‘transportType’ => ‘smtp’,
‘transportOptions’=>array(
‘host’=>’ghazalitajuddin.com’,
//’encryption’=>’tls’,
‘username’=>’***@ghazalitajuddin.com’,
‘password’=>’******’,
‘port’=>25,
),
‘logging’ => true,
‘dryRun’ => false
),
…
),
[/php]
- Configure in controller
[php]</pre>
$message = new YiiMailMessage;
$message->setBody($model->message);
$message->subject = $model->subject;
$message->addTo($model->to);
$message->from = Yii::app()->params[‘adminEmail’];
Yii::app()->mail->send($message);
<pre>[/php]
- Create our model
[php]
class EmailForm extends CFormModel
{
public $email;
public $to;
public $subject;
public $message;
public $from;
/**
* Declares the validation rules.
*/
public function rules()
{
return array(
// name, email, subject and body are required
array(’email, to, subject, message’, ‘required’),
// email has to be a valid email address
array(’email’, ’email’),
// verifyCode needs to be entered correctly
//array(‘verifyCode’, ‘captcha’, ‘allowEmpty’=>!CCaptcha::checkRequirements()),
);
}
/**
* Declares customized attribute labels.
* If not declared here, an attribute would have a label that is
* the same as its name with the first letter in upper case.
*/
public function attributeLabels()
{
return array(
‘verifyCode’=>’Verification Code’,
);
}
}
[/php]
- Finally create our view
[php]
<?php
$this->pageTitle=Yii::app()->name . ‘ – Email Others’;
$this->breadcrumbs=array(
‘Email’,
);
?>
<h1>Email others</h1>
<?php if(Yii::app()->user->hasFlash(’email’)): ?>
<div class="flash-success">
<?php echo Yii::app()->user->getFlash(’email’); ?>
</div>
<?php else: ?>
<p>
If you have business inquiries or other questions, please fill out the following form to contact us. Thank you.
</p>
<div class="form">
<?php $form=$this->beginWidget(‘CActiveForm’, array(
‘id’=>’email-form’,
‘enableClientValidation’=>true,
‘clientOptions’=>array(
‘validateOnSubmit’=>true,
),
)); ?>
<p class="note">Fields with <span class="required">*</span> are required.</p>
<?php echo $form->errorSummary($model); ?>
<div class="row">
<?php echo $form->labelEx($model,’email’); ?>
<?php echo $form->textField($model,’email’); ?>
<?php echo $form->error($model,’email’); ?>
</div>
<div class="row">
<?php echo $form->labelEx($model,’to’); ?>
<?php echo $form->textField($model,’to’); ?>
<?php echo $form->error($model,’to’); ?>
</div>
<div class="row">
<?php echo $form->labelEx($model,’subject’); ?>
<?php echo $form->textField($model,’subject’,array(‘size’=>60,’maxlength’=>128)); ?>
<?php echo $form->error($model,’subject’); ?>
</div>
<div class="row">
<?php echo $form->labelEx($model,’message’); ?>
<?php echo $form->textArea($model,’message’,array(‘rows’=>6, ‘cols’=>50)); ?>
<?php echo $form->error($model,’message’); ?>
</div>
<div class="row">
<?php echo $form->labelEx($model,’from’); ?>
<?php echo $form->textArea($model,’from’); ?>
<?php echo $form->error($model,’from’); ?>
</div>
<div class="row buttons">
<?php echo CHtml::submitButton(‘Submit’); ?>
</div>
<?php $this->endWidget(); ?>
</div><!– form –>
<?php endif; ?>
[/php]