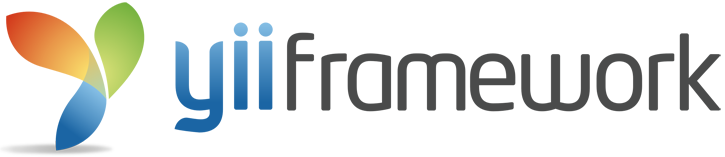
Basicly, Yii basic skeleton already come with aunthentication system which is very simple by checking username and password both admin or demo.
The default authentication files is
- UserIdentity.php
- LoginForm.php
- SiteController.php
- Login.php
- The process started when user click the login menu.
- When user click the Login menu, the SiteController will launch actionLogin() method. (SiteController.php)
- The actionLogin() method will initiate new object from LoginForm model, called $model.
- The actionLogin() method will check $_POST[‘LoginForm’] if carry data
- If $_POST[‘LoginForm’] carry data, assign $model->attributes = $_POST[‘LoginForm’]
- The new assign $model->attributes will be validate by calling $model->validate() and $model->login(). $model->login() is inherits from LoginForm class which check the user is valid or not. (LoginForm.php)
- In Login() method a new instant created,
-
$this
->_identity=
new
UserIdentity(
$this
->username,
$this
->password); (UserIdentity.php)
$this
->_identity->authenticate(); (UserIdentity.php)
- The cookie is set here too. (LoginForm.php)
- If both check on $model->attributes && $model->login() is valid, redirect to previous page.
- else is not valid, return to Login page (login.php) with error notification.
1. root/webapps/protected/components/UserIdentity.php
[php]
class UserIdentity extends CUserIdentity
{
/**
* Authenticates a user.
* The example implementation makes sure if the username and password
* are both ‘demo’.
* In practical applications, this should be changed to authenticate
* against some persistent user identity storage (e.g. database).
* @return boolean whether authentication succeeds.
*/
public function authenticate()
{
$users=array(
// username => password
‘demo’=>’demo’,
‘admin’=>’admin’,
);
if(!isset($users[$this->username]))
$this->errorCode=self::ERROR_USERNAME_INVALID;
else if($users[$this->username]!==$this->password)
$this->errorCode=self::ERROR_PASSWORD_INVALID;
else
$this->errorCode=self::ERROR_NONE;
return !$this->errorCode;
}
}
[/php]
2. root/webapps/protected/model/LoginForm.php
[php]
<?php
/**
* LoginForm class.
* LoginForm is the data structure for keeping
* user login form data. It is used by the ‘login’ action of ‘SiteController’.
*/
class LoginForm extends CFormModel
{
public $username;
public $password;
public $rememberMe;
private $_identity;
/**
* Declares the validation rules.
* The rules state that username and password are required,
* and password needs to be authenticated.
*/
public function rules()
{
return array(
// username and password are required
array(‘username, password’, ‘required’),
// rememberMe needs to be a boolean
array(‘rememberMe’, ‘boolean’),
// password needs to be authenticated
array(‘password’, ‘authenticate’),
);
}
/**
* Declares attribute labels.
*/
public function attributeLabels()
{
return array(
‘rememberMe’=>’Remember me next time’,
);
}
/**
* Authenticates the password.
* This is the ‘authenticate’ validator as declared in rules().
*/
public function authenticate($attribute,$params)
{
if(!$this->hasErrors())
{
$this->_identity=new UserIdentity($this->username,$this->password);
if(!$this->_identity->authenticate())
$this->addError(‘password’,’Incorrect username or password.’);
}
}
/**
* Logs in the user using the given username and password in the model.
* @return boolean whether login is successful
*/
public function login()
{
if($this->_identity===null)
{
$this->_identity=new UserIdentity($this->username,$this->password);
$this->_identity->authenticate();
}
if($this->_identity->errorCode===UserIdentity::ERROR_NONE)
{
$duration=$this->rememberMe ? 3600*24*30 : 0; // 30 days
Yii::app()->user->login($this->_identity,$duration);
return true;
}
else
return false;
}
}
[/php]
3. root/webapps/protected/controllers/SiteController.php
[php]
…
/**
* Displays the login page
*/
public function actionLogin()
{
$model=new LoginForm;
// if it is ajax validation request
if(isset($_POST[‘ajax’]) && $_POST[‘ajax’]===’login-form’)
{
echo CActiveForm::validate($model);
Yii::app()->end();
}
// collect user input data
if(isset($_POST[‘LoginForm’]))
{
$model->attributes=$_POST[‘LoginForm’];
// validate user input and redirect to the previous page if valid
if($model->validate() && $model->login())
$this->redirect(Yii::app()->user->returnUrl);
}
// display the login form
$this->render(‘login’,array(‘model’=>$model));
}
…
[/php]
4. root/webapps/protected/views/site/login.php
[php]
<?php
$this->pageTitle=Yii::app()->name . ‘ – Login’;
$this->breadcrumbs=array(
‘Login’,
);
?>
<h1>Login</h1>
<p>Please fill out the following form with your login credentials:</p>
<div class="form">
<?php $form=$this->beginWidget(‘CActiveForm’, array(
‘id’=>’login-form’,
‘enableClientValidation’=>true,
‘clientOptions’=>array(
‘validateOnSubmit’=>true,
),
)); ?>
<p class="note">Fields with <span class="required">*</span> are required.</p>
<div class="row">
<?php echo $form->labelEx($model,’username’); ?>
<?php echo $form->textField($model,’username’); ?>
<?php echo $form->error($model,’username’); ?>
</div>
<div class="row">
<?php echo $form->labelEx($model,’password’); ?>
<?php echo $form->passwordField($model,’password’); ?>
<?php echo $form->error($model,’password’); ?>
<p class="hint">
Hint: You may login with <tt>demo/demo</tt> or <tt>admin/admin</tt>.
</p>
</div>
<div class="row rememberMe">
<?php echo $form->checkBox($model,’rememberMe’); ?>
<?php echo $form->label($model,’rememberMe’); ?>
<?php echo $form->error($model,’rememberMe’); ?>
</div>
<div class="row buttons">
<?php echo CHtml::submitButton(‘Login’); ?>
</div>
<?php $this->endWidget(); ?>
</div><!– form –>
[/php]
Thank you in advance to the assist!