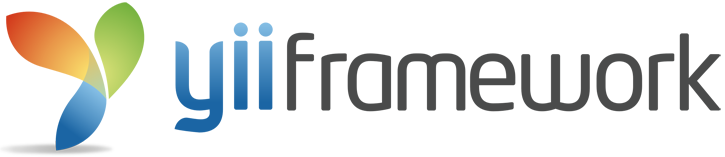
Some function is created to make code easy to read and manageable. The Lookup function is so powerfull to simplified data storage. Usually we store Approve, Not Approved, Qualified, Not Qualified in words for each records, but with Lookup class, we can make it short to an integer for each properties.
Table scheme “tbl_lookup”
[php]
CREATE TABLE tbl_lookup
(
id INTEGER NOT NULL PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(128) NOT NULL,
code INTEGER NOT NULL,
type VARCHAR(128) NOT NULL,
position INTEGER NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
[/php]
Insert some records like this
[php]
INSERT INTO tbl_lookup (name, type, code, position) VALUES (‘Activated’, ‘ActivationStatus’, 1, 1);
INSERT INTO tbl_lookup (name, type, code, position) VALUES (‘Not Activated’, ‘ActivationStatus’, 2, 2);
INSERT INTO tbl_lookup (name, type, code, position) VALUES (‘Pending Approval’, ‘membership_status’, 1, 1);
INSERT INTO tbl_lookup (name, type, code, position) VALUES (‘Approved’, ‘membership_status’, 2, 2);
INSERT INTO tbl_lookup (name, type, code, position) VALUES (‘Not Approved’, ‘membership_status’, 3, 3);
[/php]
Class Lookup.php
[php]
<?php
class Lookup extends CActiveRecord
{
/**
* The followings are the available columns in table ‘tbl_lookup’:
* @var integer $id
* @var string $object_type
* @var integer $code
* @var string $name_en
* @var string $name_fr
* @var integer $sequence
* @var integer $status
*/
private static $_items=array();
/**
* Returns the static model of the specified AR class.
* @return CActiveRecord the static model class
*/
public static function model($className=__CLASS__)
{
return parent::model($className);
}
/**
* @return string the associated database table name
*/
public function tableName()
{
return ‘{{lookup}}’;
}
/**
* Returns the items for the specified type.
* @param string item type (e.g. ‘PostStatus’).
* @return array item names indexed by item code. The items are order by their position values.
* An empty array is returned if the item type does not exist.
*/
public static function items($type)
{
if(!isset(self::$_items[$type]))
self::loadItems($type);
return self::$_items[$type];
}
/**
* Returns the item name for the specified type and code.
* @param string the item type (e.g. ‘PostStatus’).
* @param integer the item code (corresponding to the ‘code’ column value)
* @return string the item name for the specified the code. False is returned if the item type or code does not exist.
*/
public static function item($type,$code)
{
if(!isset(self::$_items[$type]))
self::loadItems($type);
return isset(self::$_items[$type][$code]) ? self::$_items[$type][$code] : false;
}
/**
* Loads the lookup items for the specified type from the database.
* @param string the item type
*/
private static function loadItems($type)
{
self::$_items[$type]=array();
$models=self::model()->findAll(array(
‘condition’=>’type=:type’,
‘params’=>array(‘:type’=>$type),
‘order’=>’position’,
));
foreach($models as $model)
self::$_items[$type][$model->code]=$model->name;
}
}
[/php]
In User.php class just add
[php]
class User extends CActiveRecord
{
const STATUS_ACTIVATED=1;
const STATUS_NOT_ACTIVATED=2;
…
[/php]
And finally at my list view
[php]
<b><?php echo CHtml::encode($data->getAttributeLabel(‘activationstatus’)); ?>:</b>
<?php echo Lookup::item(‘ActivationStatus’,$data->activationstatus); ?>
[/php]
Another example
[php]
<?php echo $form->dropDownList($model,’membership_status’,Lookup::items(‘membership_status’)); ?>
[/php]
And another one
[php]
<?php echo $form->dropDownList($model,’user_id’, CHtml::listData( User::model()->findAll(),’id’,’username’));
[/php]
Leave a Reply